Published
- 6 min read
Entity Framework: OnDelete Behavior
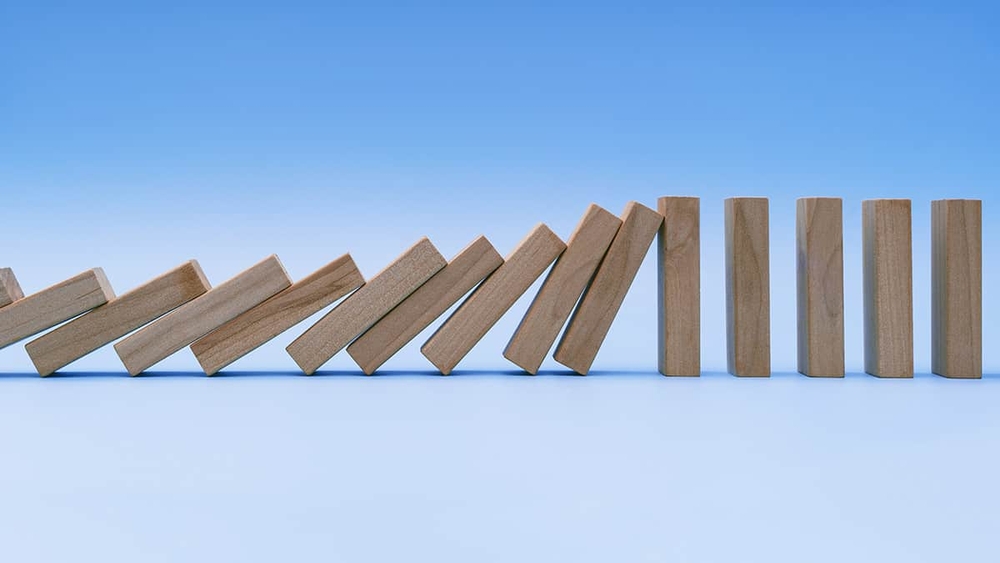
When building applications with Entity Framework, one of the most critical aspects of data management is handling the deletion of related entities. How should your application behave when you delete a customer who has active orders? What happens to a user’s posts when their account is removed? These scenarios are where Entity Framework’s OnDelete behavior comes into play.
Understanding Entity Relationships
Before diving into OnDelete behaviors, let’s establish some fundamental concepts.
A database relationship typically involves two entities:
- A Principal (or parent) entity that contains the primary key
- A Dependent (or child) entity that contains the foreign key
For example, in a blog application:
- A
User
would be a principal entity - Their
Posts
would be dependent entities - Each
Post
has a foreign key referencing theUser
’s primary key - Both entities can have navigation properties to access related data
The Challenge of Deleting Related Entities
When you delete a principal entity, you face two main scenarios:
- Deleting the principal: What happens to all dependent entities?
- Severing relationships: What occurs when you break the connection between principal and dependent entities?
This is where Entity Framework’s OnDelete behavior provides various strategies to handle these scenarios safely and efficiently.
How Entity Framework Tracks Changes
Before diving into OnDelete behaviors, it’s crucial to understand how Entity Framework tracks changes.
Change Tracking Context
Entity Framework maintains a “change tracking context” that monitors all entities retrieved through the DbContext. This tracking works by reference, meaning:
- When you load an entity from the database, EF keeps a reference to that object
- Any modifications to the object’s properties are detected by comparing current values with original values
- When you call
Remove()
on an entity, EF marks it for deletion in the change tracker - Nothing is actually sent to the database until you call
SaveChanges()
For example:
// This only marks the user for deletion in memory
context.Users.Remove(user);
// At this point, EF generates and executes the DELETE SQL command
await context.SaveChanges();
This delayed execution pattern allows you to:
- Batch multiple operations together
- Validate changes before they hit the database
- Roll back changes if something goes wrong
- Implement unit of work pattern effectively
Reference Tracking and Navigation Properties
Entity Framework’s change tracker handles navigation properties and collections in specific ways that are important to understand:
var user = context.Users
.Include(u => u.Posts)
.FirstOrDefault(u => u.Id == 1);
// This only removes references and sets FKs to null if the relationship is optional
// It does NOT mark entities for deletion
user.Posts.Clear();
// To actually delete the posts, you need to explicitly remove them:
context.RemoveRange(user.Posts);
// Or remove them one by one:
foreach(var post in user.Posts.ToList())
{
context.Posts.Remove(post);
}
When working with navigation properties, it’s crucial to understand that:
- Clear() method only breaks the relationships between entities
- For optional relationships (nullable foreign keys), it sets the foreign keys to null
- For required relationships (non-nullable foreign keys), Clear() will throw an exception
- To delete dependent entities, you must explicitly call Remove() or RemoveRange()
- Entity Framework will track all these changes and apply them when SaveChanges() is called
This behavior ensures that you have explicit control over whether you want to merely disconnect entities or actually delete them from the database.
OnDelete Behaviors Explained
Entity Framework offers several OnDelete behaviors, each serving different use cases.
Database-Side Behaviors
- Cascade
- Automatically deletes dependent entities when the principal is deleted
- Executed directly by the database
- Best for required relationships where dependents can’t exist without their principal
- Restrict
- Prevents deletion of the principal if dependent entities exist
- Useful when you want to enforce data integrity explicitly
- SetNull
- Sets the foreign key to null when the principal is deleted
- Only works with optional relationships (nullable foreign keys)
- Preserves dependent entities while removing the relationship
- NoAction
- Leaves dependent entities unchanged
- May cause referential integrity violations if not handled properly
Client-Side Behaviors
- ClientCascade
- Similar to Cascade, but executed by Entity Framework
- Only affects entities tracked by the current DbContext
- Requires loading dependent entities into memory
- ClientSetNull
- Sets foreign keys to null in memory
- Changes aren’t saved until SaveChanges() is called
- Requires loading dependent entities
- ClientNoAction
- No automatic handling by Entity Framework
- Developers must manage relationships manually
Database Compatibility
Database-Specific Limitations
Not all databases support the same deletion behaviors:
- SQL Server
- Doesn’t support cascade delete paths longer than one level
- May require client-side cascade for complex hierarchies
- Has limitations with circular references
- PostgreSQL
- Supports multi-level cascade deletes
- Handles circular references well
- More flexible with deletion strategies
- SQLite
- Limited support for complex referential actions
- May require client-side handling
When your database doesn’t support certain deletion behaviors, you have several options:
- Use client-side behaviors (ClientCascade)
- Implement manual deletion logic
- Use a combination of database and client-side strategies
Example: Handling SQL Server Limitations
// When cascade delete isn't possible in SQL Server
public class BloggingContext : DbContext
{
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity < Post > ()
.HasOne(p => p.Blog)
.WithMany(b => b.Posts)
.OnDelete(DeleteBehavior.ClientCascade);
}
}
Choosing the Right Behavior
Your choice of OnDelete behavior should depend on several factors.
For Required Relationships
- Use Cascade when dependents should always be deleted with their principal
- Choose Restrict when you want to prevent accidental deletions
- Avoid SetNull as it’s incompatible with required relationships
For Optional Relationships
- Use SetNull when dependents should survive principal deletion
- Choose ClientSetNull when you need more control over the nulling process
- Use Cascade when dependents should still be deleted despite optional relationship
Performance Considerations
The choice of OnDelete behavior can significantly impact your application’s performance:
- Database-side behaviors (Cascade, Restrict, SetNull)
- More efficient for large datasets
- Don’t require loading entities into memory
- Better for maintaining data consistency
- Client-side behaviors (ClientCascade, ClientSetNull)
- Require loading dependent entities
- Provide more control and flexibility
- Better for complex business logic
- May impact performance with large datasets
Common Pitfalls and Solutions
Handling Circular References
When dealing with self-referencing or circular relationships (like hierarchical data):
- Use client-side behaviors to break deletion cycles
- Consider implementing custom deletion logic
- Be mindful of the order of operations when saving changes
Loading States
Remember that client-side behaviors require entities to be loaded:
// This won't work with ClientCascade if posts aren't loaded
context.Users.Remove(user);
// This will work properly
var userWithPosts = context.Users
.Include(u => u.Posts)
.FirstOrDefault(u => u.Id == userId);
context.Users.Remove(userWithPosts);
Best Practices
- Document your choices: Make OnDelete behavior decisions explicit in your code and documentation
- Consider data volume: Choose database-side behaviors for large datasets
- Think about maintenance: Use client-side behaviors when you need more control or have complex business rules
- Test thoroughly: Verify deletion behavior with unit tests and integration tests
- Monitor performance: Watch for N+1 query problems when using client-side behaviors
Conclusion
Understanding and properly configuring Entity Framework’s OnDelete behavior is crucial for building robust applications. Take time to evaluate your specific needs, considering factors like data relationships, performance requirements, and business rules. The right configuration will lead to cleaner code, better performance, and fewer data integrity issues.
Remember: There’s no one-size-fits-all solution. The best approach depends on your specific use case, performance requirements, and business rules. Regular testing and monitoring will help ensure your chosen strategy continues to meet your application’s needs as it grows.